IronSource - iOS
Based on the IronSource demo project: https://github.com/ironsource-mobile/Mediation-Demo-Apps/tree/master/iOS/Swift you can check the Nefta custom adapter integration example here: https://github.com/Nefta-io/NeftaISAdapter:
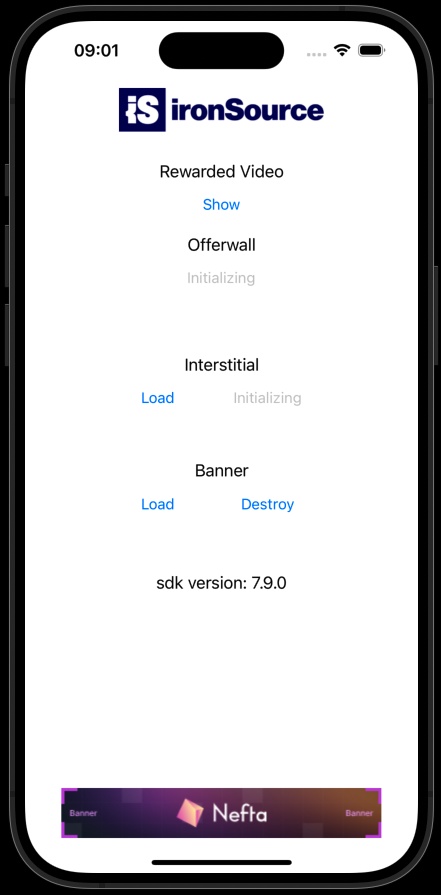
Include the SDK
You can download the latest Nefta adapter from https://github.com/Nefta-io/NeftaISAdapter/releases.
Extract the content of the zip file to your project.
Given that your project name is NeftaISAdapter it could look like this:
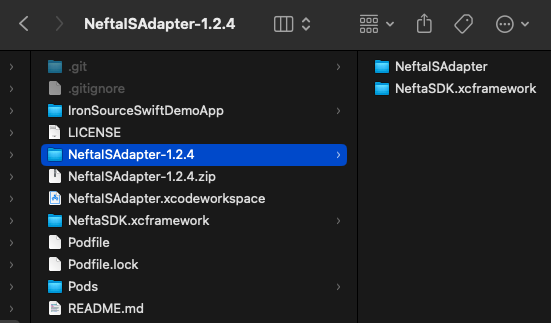
In project settings add NeftaSDK.xcframework to Frameworks, Libraries, and Embedded Content for the project target:
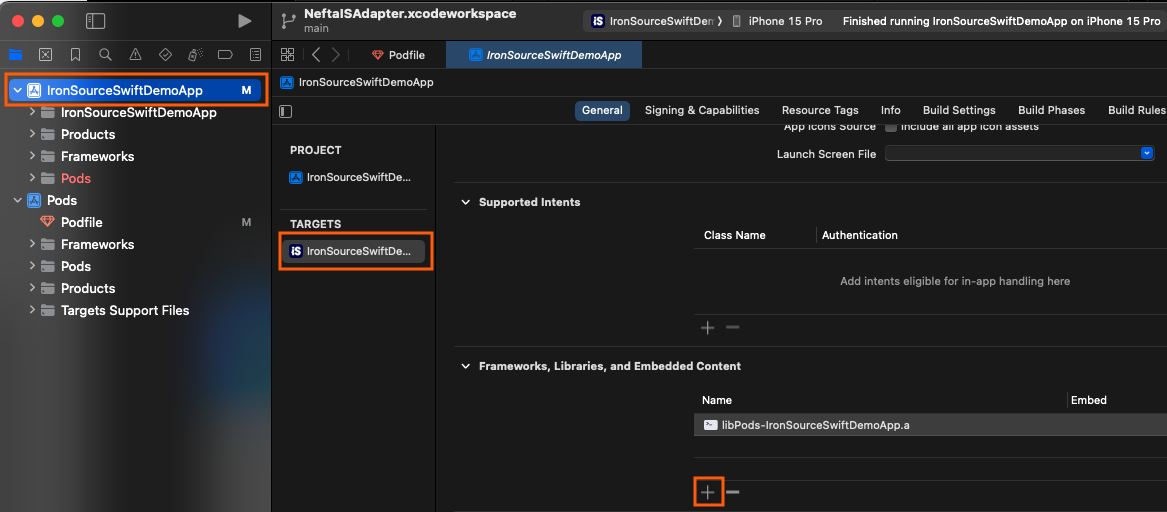
In the opened popup click on Add Other... and select NeftaSDK.xcframework in the extracted location from the previous step. After this, the NeftaSDK should be included like this:
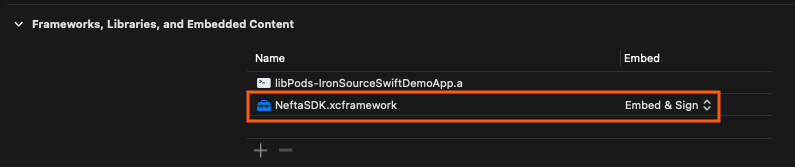
The last step is to include the adapter source code. We can do this by right-clicking on the project and selecting Add Files to "YourProject"...:
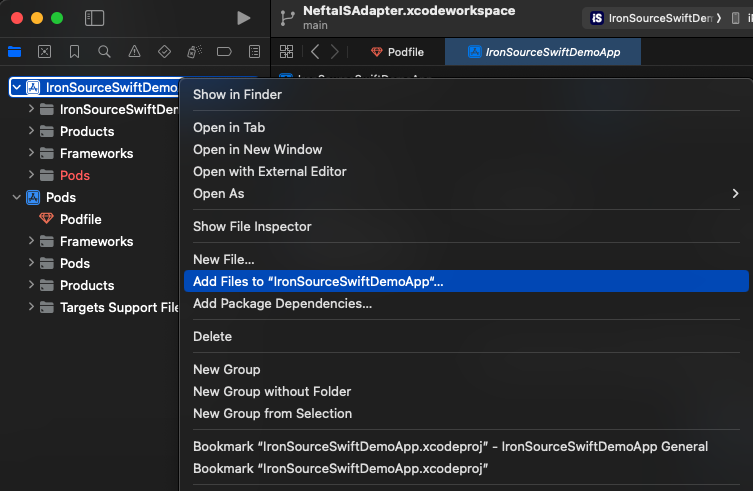
Select NeftaISAdapter from the extracted folder and make sure to add this folder with Create groups and not Create folder references and that the files will be added as targets to your project:
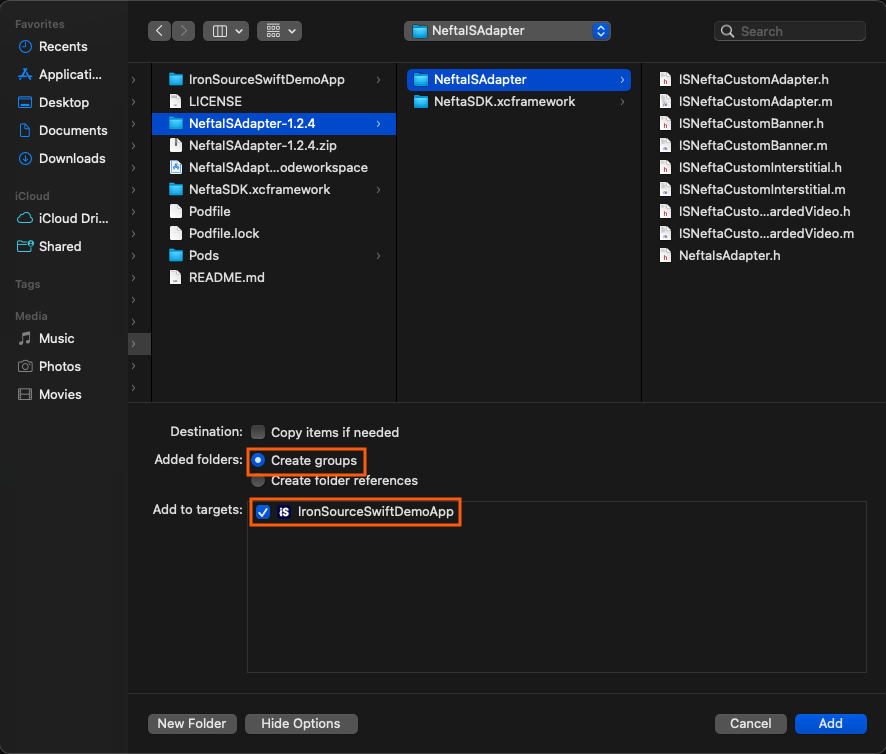
Make sure to check the requires full screen permission for best experience (during orientation switches on iPads and such):
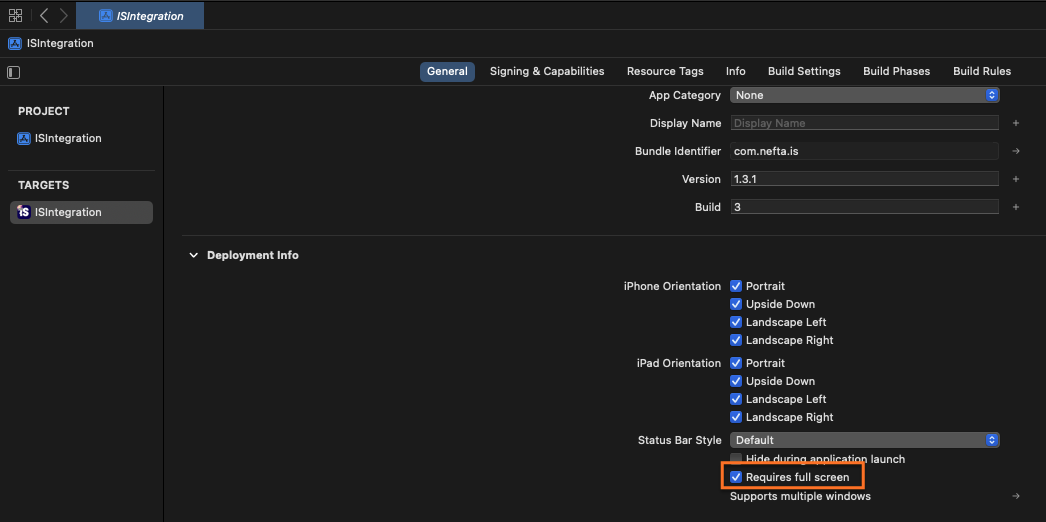
Code integration
The plugin has to be manually initialized, preferably as soon as possible to have good game events:
import NeftaSDK
let plugin = NeftaPlugin.Init(appId: "5661184053215232")
#import "NeftaAdapter.h"
NeftaPlugin *plugin = [NeftaPlugin InitWithAppId: @"5661184053215232"];
In the dashboard you can access the AppId which you need to enter in the code above.
Events
Game events can be then recorded as follows:
plugin.Events.AddSpendEvent(category: NeftaEvents.ResourceCategory.PremiumCurrency,
method: NeftaEvents.SpendMethod.Upgrade)
plugin.Events.AddProgressionEvent(status: NeftaEvents.ProgressionStatus.Start,
type: NeftaEvents.ProgressionType.GameplayUnit,
source: NeftaEvents.ProgressionSource.CoreContent,
name: "area-4",
value: 21)
[plugin.Events AddSpendEventWithCategory: ResourceCategoryPremiumCurrency
method: SpendMethodUpgrade];
[plugin.Events AddProgressionEventWithStatus: ProgressionStatusStart
type: ProgressionTypeGameplayUnit
source: ProgressionSourceCoreContent
name: "area-4"
value: 21];
Testing
To put the adapter in test mode and enable logs:
NeftaPlugin.EnableLogging(enable: true)
[NeftaPlugin EnableLogging: true];
For testing ads, you can retrieve the Nefta identifier from the device with:
let nuid = plugin.ShowNuid()
NSString *nuid = [plugin ShowNuid];
With this, you can set custom ad behavior in the dashboard.
All done
With this technical part, make sure you have Nefta mediation enabled in your LevelPlay configuration.
Updated 2 months ago