Android
Sample project with example integration can be downloaded from: https://github.com/Nefta-io/NeftaSDK-Android:
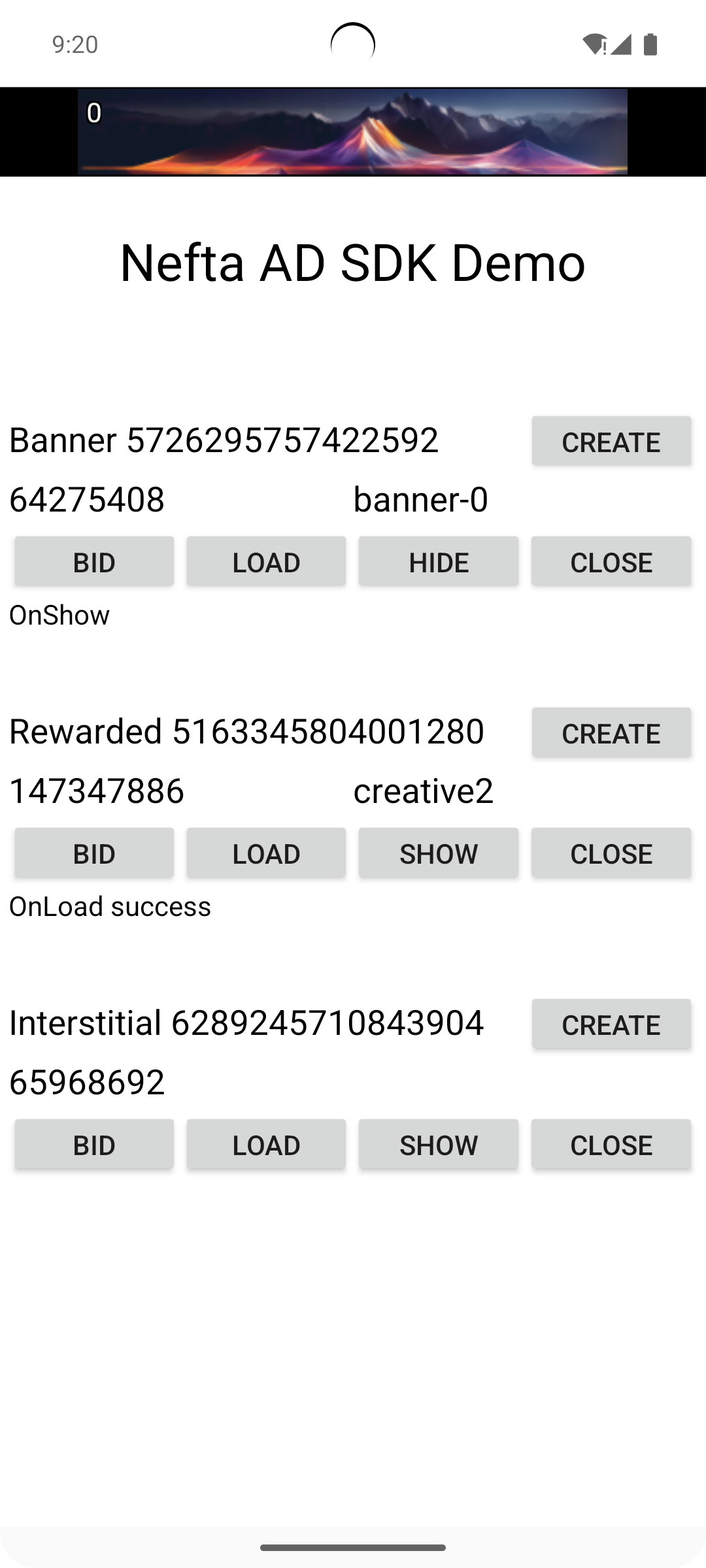
Requirements
Minimal Android version 4.4 (API level 19)
Include the SDK
You can download the latest NeftaPlugin module from: https://github.com/Nefta-io/NeftaSDK-Android/releases.
Extract the NeftaPugin-release.aar file and include it in your Android project in /libs folder, so that it will look something like this:
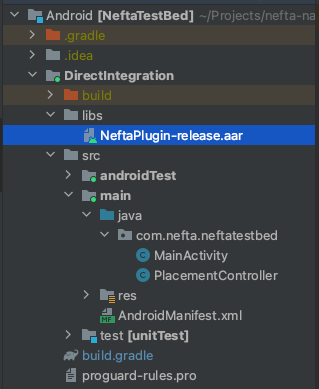
Then include this module as a dependency in your project build.gradle:
implementation files('libs/NeftaPlugin-release.aar')
Permissions
For the SDK to work it requires permissions for the network which you need to add to your project AndroidManifest.xml:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
Configuration
To serve and track the ads for your app, you have to configure ad units in the dashboard. There you will get the application ID for the next step.
Code integration
You initialize the SDK with the following code:
import com.nefta.sdk.NeftaPlugin;
NeftaPlugin plugin = NeftaPlugin.Init(getApplicationContext(), "yourAppId");
Do this as soon as possible in the application startup, to ensure the accurate event recording. If you leave the appId parameter null or empty the SDK will run in demo mode. This means that it'll always show dummy ads. To test the native integration without dashboard configuration.
If you want just the events this is all that is needed. You can proceed to the implementation of the actual events.
Code integration - Ads
To start the ad logic and retrieve all the configuration (so that no resources are wasted in case you are not having ads or you don't want them straight away):
_plugin.EnableAds(true);
Since the SDK doesn't want to introduce its own lifecycle dependencies, you need to call OnResume and OnPause methods when the app goes or comes from the background:
@Override
protected void onResume() {
super.onResume();
_plugin.OnResume();
}
@Override
protected void onPause() {
super.onPause();
_plugin.OnPause();
}
Banner
To show banners create an instance of NBanner like this:
NBanner banner = new NBanner("adUnitId", bannerViewParent);
// or
NBanner banner = new NBanner("adUnitId", NBanner.Position.TOP);
banner._listener = new NBannerListener() {
@Override
public void OnLoadFail(NAd ad, NError error) {
}
@Override
public void OnLoad(NAd ad, int width, int height) {
}
@Override
public void OnShowFail(NAd ad, NError error) {
}
@Override
public void OnShow(NAd ad) {
}
@Override
public void OnClose(NAd ad) {
}
};
Auto flow
To load and show the banner you then call:
banner.SetAutoRefresh(true);
banner.Show();
With this the banner will refresh automatically ensuring you to maximize revenue.
Or if you want to control each banner flow cycle manually:
banner.Load();
// when the ad is loaded (after receiving OnLoad callback or when banner.CanShow() returns true)
banner.Show();
Interstitial
To load and show interstitial do:
NInterstitial interstitial = new NInterstitial("interstitialAdUnitId");
interstitial._listener = new NInterstitialListener() {
@Override
public void OnLoadFail(NAd ad, NError error) {
}
@Override
public void OnLoad(NAd ad, int width, int height) {
}
@Override
public void OnShowFail(NAd ad, NError error) {
}
@Override
public void OnShow(NAd ad) {
}
@Override
public void OnClose(NAd ad) {
}
};
interstitial.Load();
// when the ad loads (after receiving OnLoad callback or when interstitial.CanShow() returns true)
interstitial.Show();
Rewarded
To load and show rewarded video do:
NRewarded rewarded = new NRewarded("rewardedAdUnitId");
rewarded._listener = new NRewardedListener() {
@Override
public void OnLoadFail(NAd ad, NError error) {
}
@Override
public void OnLoad(NAd ad, int width, int height) {
}
@Override
public void OnShowFail(NAd ad, NError error) {
}
@Override
public void OnShow(NAd ad) {
}
@Override
public void OnClose(NAd ad) {
}
@Override
public void OnReward(NAd ad) {
}
};
rewarded.Load();
// when the ad loads (after receiving OnLoad callback or when rewarded.CanShow() returns true)
rewarded.Show();
Bidding
In case you only want to load an ad if it has specific price you can just bid on specific adUnit and decide if you want to proceed with that bid, for example:
NRewarded rewarded = new NRewarded(_placement._id);
rewarded._listener = new NRewardedListener() {
@Override
public void OnBid(NAd ad, BidResponse bidResponse, NError error) {
if (bidResponse != null && bidResponse._price > 0.42) {
rewarded.Load();
}
}
};
rewarded.Bid();
You can verify the correct SDK behaviour through logs: Testing
Updated 2 months ago